Adding an Emoji Picker to Your Rails 8 App with ActionText
Introduction
If you're building a Rails app that involves rich text editing, like comments, messages, or posts, chances are users will appreciate an easy way to insert emojis. Emojis enhance communication, making interactions more engaging and expressive. In this guide, we’ll walk you through integrating an emoji picker into a Rails app using ActionText and emoji-picker-element, a lightweight and modern emoji picker.
Step 1: Install and Configure emoji-picker-element
First, install the emoji picker package using Importmap in your Rails app:
bin/importmap pin emoji-picker-element
This makes the emoji-picker web component available in your app. Your config/importmap.rb
file should now include the pinned emoji picker:
# Pin npm packages by running ./bin/importmap
pin "application"
pin "@hotwired/turbo-rails", to: "turbo.min.js"
pin "@hotwired/stimulus", to: "stimulus.min.js"
pin "@hotwired/stimulus-loading", to: "stimulus-loading.js"
pin_all_from "app/javascript/controllers", under: "controllers"
pin "trix"
pin "@rails/actiontext", to: "actiontext.esm.js"
pin "emoji-picker-element", to: "https://cdn.jsdelivr.net/npm/emoji-picker-element@^1/index.js"
Step 2: Add an Emoji Picker to Your ActionText Editor
We're assuming you've already set up ActionText in your project. That means you've used the Rails generator to install it and connected it to the model you want to use it with. For this example, we have a Post
model which uses ActionText.
class Post < ApplicationRecord
has_rich_text :content
end
First, in our post_form.html.erb
, we add a Stimulus target to the content field.
<%= form_with model: @post do |f| %>
<%= form.label :content %>
<%= form.rich_text_area :content, data: {post_target: 'trixEditor'} %>
<%= f.submit "Save Post" %>
<% end %>
Step 3: Add JavaScript to Add Emoji Button and Handle Emoji Selection
We'll add an emoji button to the ActionText toolbar, allowing users to insert emojis at the cursor position. Since we already have a Stimulus controller for Post
, we'll use Stimulus targets to interact with the trixEditor
. Next, we'll select the ActionText toolbar using the following approach:
let trixblockTools = document.querySelector("[data-trix-button-group=block-tools]");
Next, we'll create an emoji button to add to the toolbar. Then, we'll initialize the emoji picker. When a user picks an emoji from the picker, an event called emoji-click
is triggered. This event provides a detail
object containing all the necessary information about the selected emoji.
{
"emoji": {
"annotation": "grinning face with big eyes",
"group": 0,
"order": 2,
"tags": ["awesome", "big"],
"unicode": "😃",
"version": 0.6,
"shortcodes": ["grinning_face_with_big_eyes", "smiley"]
},
"skinTone": 0,
"unicode": "😃"
}
Once the event is captured, we'll use the insertString
method of TrixEditor to insert the emoji from the detail
object. After the emoji is selected, we'll remove the picker element. The complete controller code will look like this:
import { Controller } from "@hotwired/stimulus";
import { Picker } from "emoji-picker-element";
// Connects to data-controller="post"
export default class extends Controller {
static targets = ["trixEditor"];
connect() {
this.addEmojiButton();
}
addEmojiButton() {
let trixToolbar = document.querySelector("[data-trix-button-group=block-tools]");
if (trixToolbar) {
let emojiButton = this.createEmojiButton();
trixToolbar.prepend(emojiButton);
}
}
createEmojiButton() {
let button = document.createElement("button");
button.type = "button";
button.className = "trix-button";
button.innerHTML = "😀";
button.title = "Insert Emoji";
button.tabIndex = "-1";
button.setAttribute("data-action", "click->post#insertEmoji");
return button;
}
insertEmoji() {
let picker = this.initializeEmojiPicker();
document.body.appendChild(picker);
picker.addEventListener("emoji-click", (event) => {
if (this.trixEditorTarget) {
this.trixEditorTarget.editor.insertString(event.detail.unicode);
picker.remove(); // Remove picker after selection
}
});
}
initializeEmojiPicker() {
let picker = new Picker();
picker.style.position = "absolute";
picker.style.top = "80px";
picker.style.left = "415px";
picker.style.boxShadow = "0 4px 8px rgba(0, 0, 0, 0.2)";
picker.classList.add("light");
return picker;
}
}
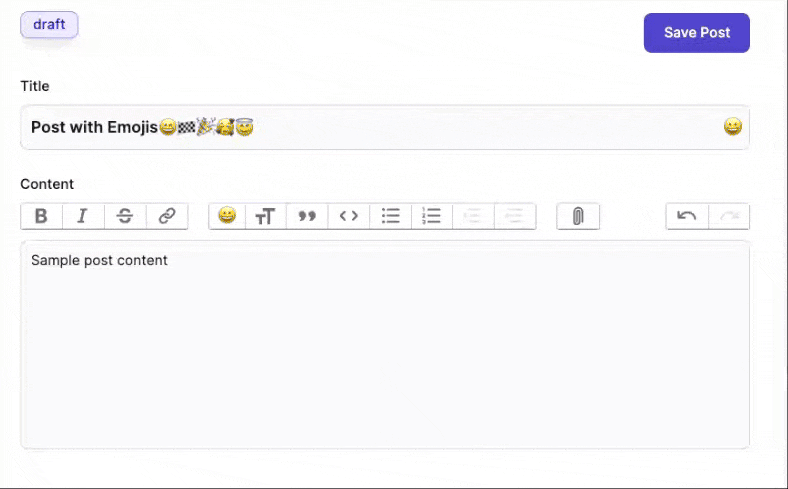
Wrapping Up
Adding an emoji picker to your Rails app with ActionText is a great way to enhance user experience. With emoji-picker-element, the process is simple, efficient, and highly customizable. At Bytecific, we specialize in building high-performance Rails applications. Contact us today to bring your ideas to life!